【教學目的】
我們要讀入如下的資料,此資料檔以逗點分隔資料,我們要將資料一行一行讀出來(放在一個字串),並且以逗點方式將資料分開(字串陣列)。
【第一個畫面】未分割資料
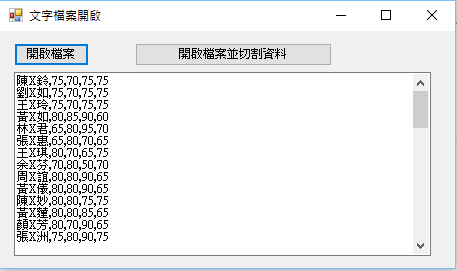
【第二個畫面】分割資料
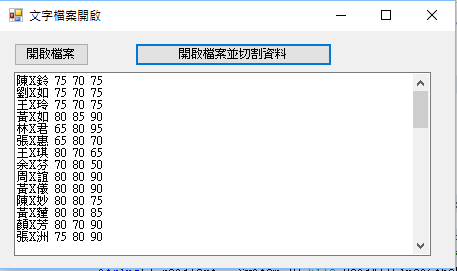
陳X鈴,75,70,75,75
劉X如,75,70,75,75
王X玲,75,70,75,75
黃X如,80,85,90,60
林X君,65,80,95,70
張X惠,65,80,70,65
王X琪,80,70,65,75
余X芬,70,80,50,70
周X誼,80,80,90,65
黃X儀,80,80,90,65
陳X妙,80,80,75,75
黃X蓮,80,80,85,65
顏X芳,80,70,90,65
張X洲,75,80,90,75
王X聰,65,70,75,45
胡X虹,20,80,70,65
~~以下 省略~~
【程式碼】
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApplication2
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
tbResult.Text = "";
// 建立一個OpenFileDialog物件
OpenFileDialog openFileDialog1 = new OpenFileDialog();
// 設定OpenFileDialog屬性
openFileDialog1.Title = "選擇要開啟的CSV檔案";
openFileDialog1.Filter = "CSV Files (.csv)|*.csv|All Files (*.*)|*.*";
openFileDialog1.FilterIndex = 1;
openFileDialog1.Multiselect = true;
// 喚用ShowDialog方法,打開對話方塊
if (openFileDialog1.ShowDialog() == System.Windows.Forms.DialogResult.OK)
{
string theFile = openFileDialog1.FileName; //取得檔名
Encoding enc = Encoding.GetEncoding("big5"); //設定檔案的編碼
string[] readText = System.IO.File.ReadAllLines(theFile, enc); //以指定的編碼方式讀取檔案
foreach (string s in readText)
{
tbResult.Text += s + "\r\n";
}
}
}
private void button2_Click(object sender, EventArgs e)
{
tbResult.Text = "";
// 建立一個OpenFileDialog物件
OpenFileDialog openFileDialog1 = new OpenFileDialog();
// 設定OpenFileDialog屬性
openFileDialog1.Title = "選擇要開啟的CSV檔案";
openFileDialog1.Filter = "CSV Files (.csv)|*.csv|All Files (*.*)|*.*";
openFileDialog1.FilterIndex = 1;
openFileDialog1.Multiselect = true;
// 喚用ShowDialog方法,打開對話方塊
if (openFileDialog1.ShowDialog() == System.Windows.Forms.DialogResult.OK)
{
string theFile = openFileDialog1.FileName; //取得檔名
Encoding enc = Encoding.GetEncoding("big5"); //設定檔案的編碼
string[] readText = System.IO.File.ReadAllLines(theFile, enc); //以指定的編碼方式讀取檔案
foreach (string s in readText)
{
string[] ss = s.Split(','); //將一列的資料,以逗號的方式進行資料切割,並將資料放入一個字串陣列
tbResult.Text += ss[0] + " " + ss[1] + " " + ss[2] + " " + ss[3] + " " + ss[4] + "\r\n";
//資料分別在取出的字串陣列裏,姓名->ss[0], 成績1->ss[1], 成績2->ss[2], 成績3->ss[3], 成績4->ss[4]
}
}
}
}
}
【一維字串陣列-字串物件】
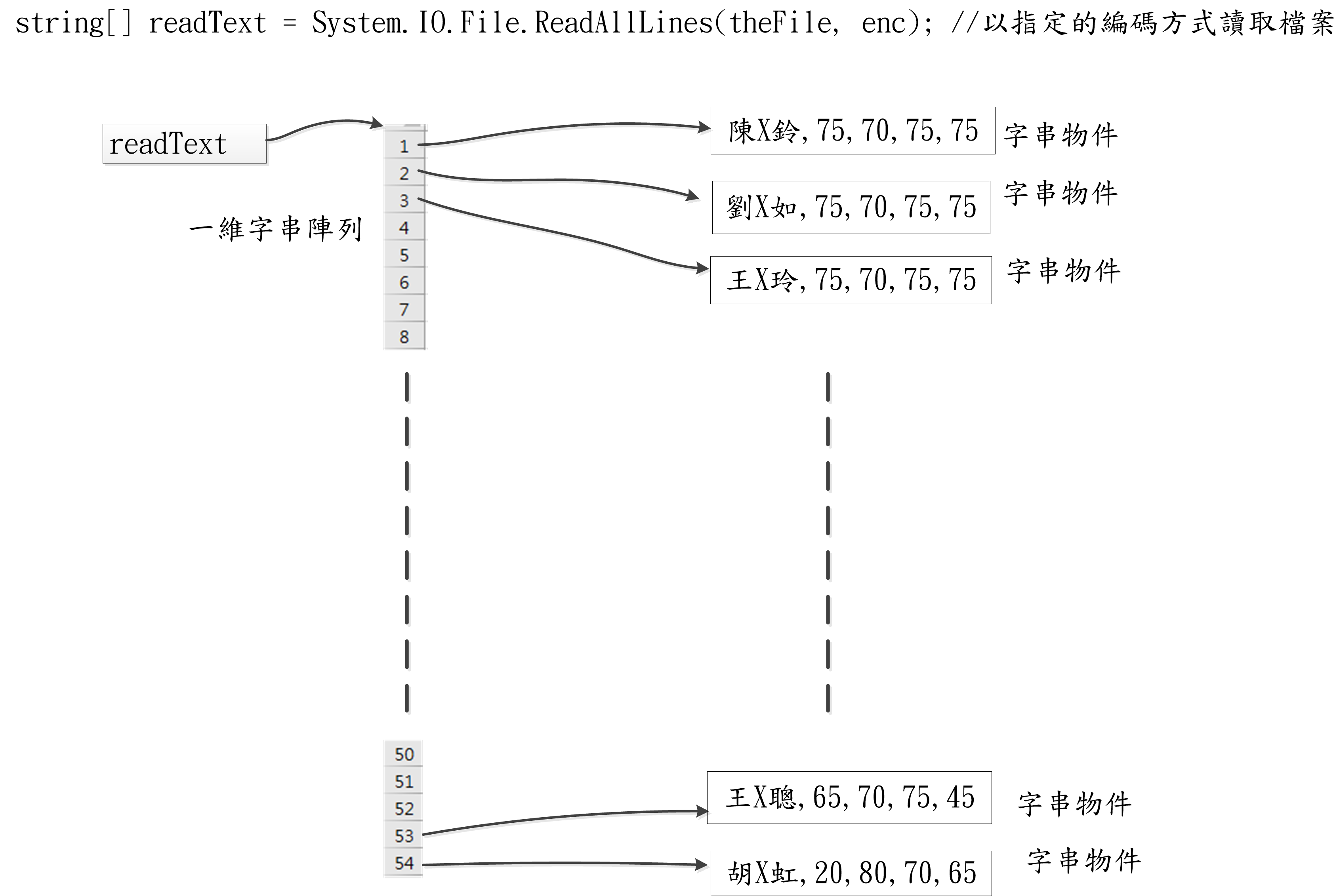
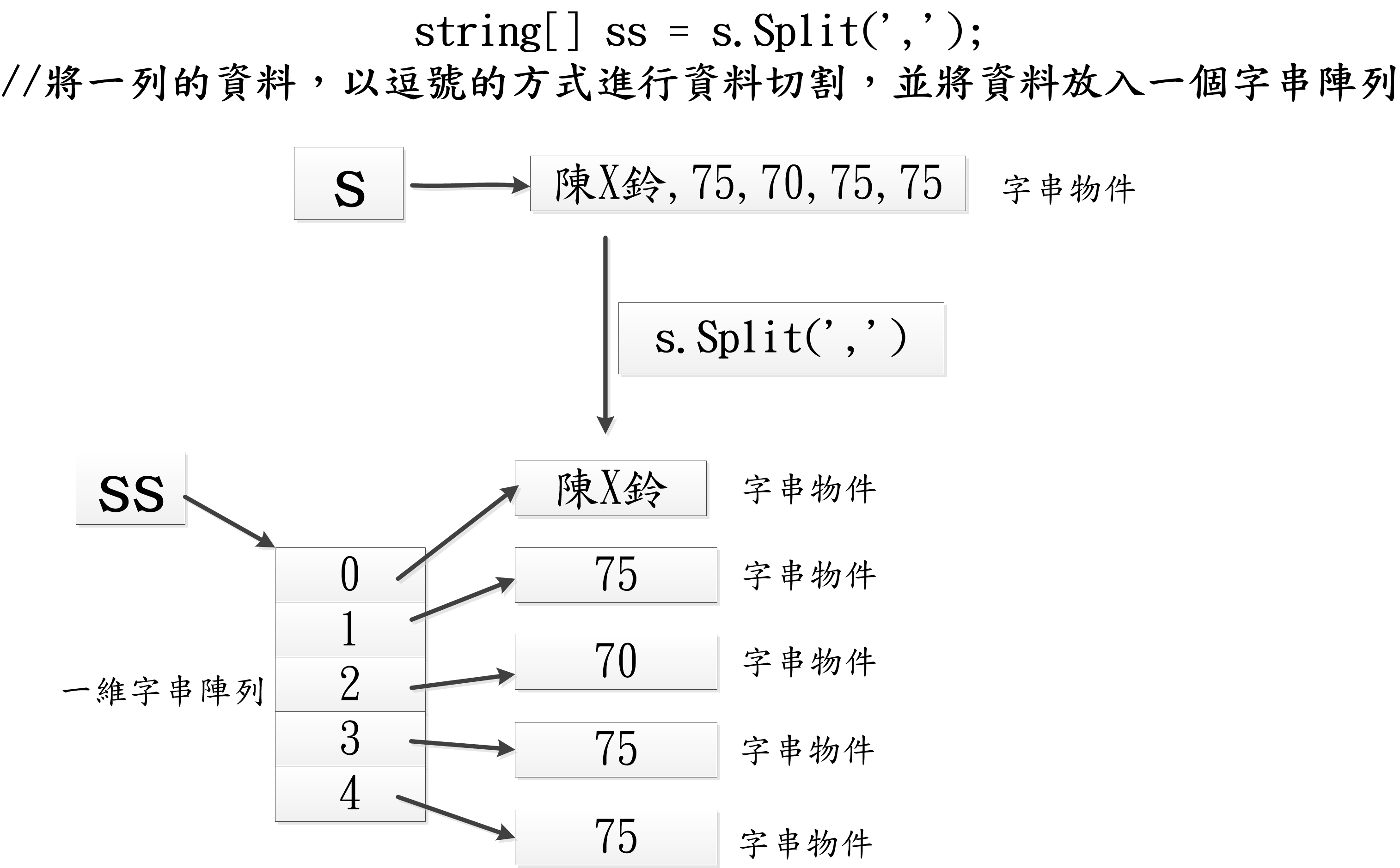
底下的改版程式係將讀出來的資料,轉成浮點數後,放入一個2維的浮點數陣列,然後,將總分加總後,放到最後一個欄位(每列)。
private void button2_Click(object sender, EventArgs e)
{
tbResult.Text = "";
// 建立一個OpenFileDialog物件
OpenFileDialog openFileDialog1 = new OpenFileDialog();
// 設定OpenFileDialog屬性
openFileDialog1.Title = "選擇要開啟的CSV檔案";
openFileDialog1.Filter = "CSV Files (.csv)|*.csv|All Files (*.*)|*.*";
openFileDialog1.FilterIndex = 1;
openFileDialog1.Multiselect = true;
// 喚用ShowDialog方法,打開對話方塊
if (openFileDialog1.ShowDialog() == System.Windows.Forms.DialogResult.OK)
{
string theFile = openFileDialog1.FileName; //取得檔名
Encoding enc = Encoding.GetEncoding("big5"); //設定檔案的編碼
string[] readText = System.IO.File.ReadAllLines(theFile, enc); //以指定的編碼方式讀取檔案
string[] name = new string[readText.Length];//宣告一個1維字串陣列,來儲存所有的姓名
double[][] allData = new double[readText.Length][4]; //宣告一個2維double陣列,用來儲存所有的成績資料,第一維的大小是資料的列數(筆數)
int line = 0; //表第幾行(第幾列,每一列為一個學生的資料)
foreach (string s in readText)
{
string[] ss = s.Split(','); //將一列的資料,以逗號的方式進行資料切割,並將資料放入一個字串陣列
name[line] = ss[0]; //切出來的字串,第0個元素是姓名
allData[line][0] = double.Parse(ss[1]);
allData[line][1] = double.Parse(ss[2]);
allData[line][2] = double.Parse(ss[3]);
allData[line][3] = double.Parse(ss[4]);
allData[line][4] = allData[line][0] + allData[line][1] + allData[line][2]+ allData[line][3]; //將每個人的成績加起來放在最後一欄
tbResult.Text += name[line] + " " + allData[line][0] + " " + allData[line][1] + " " + allData[line][2] + " " + allData[line][3] + " " + allData[line][4] + "\r\n";
line++; //進行下一筆資料的處理
//資料分別在取出的字串陣列裏,姓名->ss[0], 成績1->ss[1], 成績2->ss[2], 成績3->ss[3], 成績4->ss[4]
}
}
}