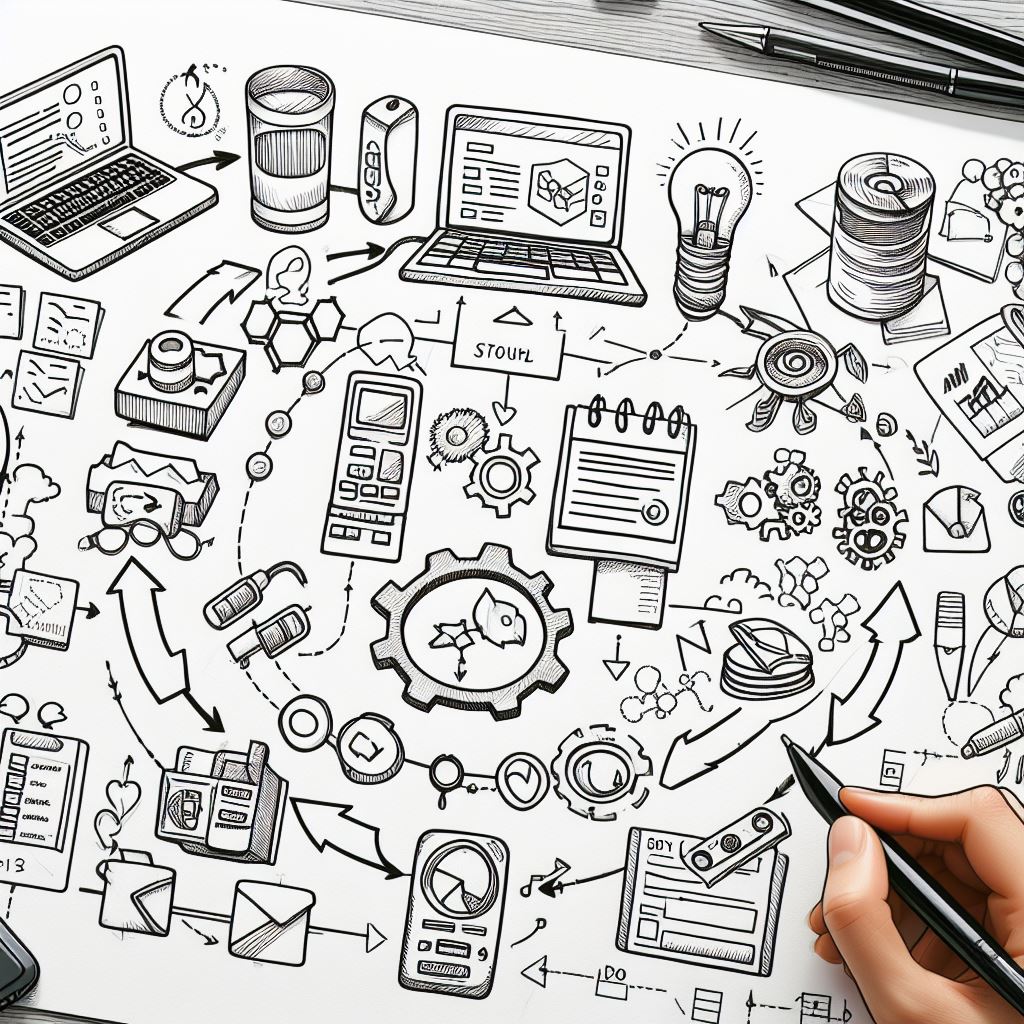
Creating an Interactive Virtual Dressing Room with OpenCV
Creating an interactive virtual dressing room using OpenCV involves a series of steps that include image processing, object detection, and augmentation. Here’s a detailed guide on how to create a basic version of a virtual dressing room:
Prerequisites
- Python installed on your machine.
- OpenCV and numpy libraries for image processing.
- dlib library for facial landmarks detection (optional but useful for precise positioning).
Step-by-Step Implementation
1. Install Required Libraries
First, install the necessary libraries using pip:
pip install numpy opencv-python dlib
2. Load the Necessary Models and Resources
For facial landmark detection, you need a pre-trained model provided by dlib:
import cv2
import numpy as np
import dlib
# Load dlib's pre-trained face detector model and the facial landmarks predictor model
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor('shape_predictor_68_face_landmarks.dat')
3. Detect Facial Landmarks
Define a function to detect facial landmarks which will help position the clothing items accurately:
def get_landmarks(image):
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
faces = detector(gray)
if len(faces) > 0:
landmarks = predictor(gray, faces[0])
return [(p.x, p.y) for p in landmarks.parts()]
return []
4. Load and Prepare Clothing Image
Load the clothing image and prepare it for overlaying onto the body:
def overlay_image(background, overlay, position):
bg_height, bg_width = background.shape[:2]
overlay_height, overlay_width = overlay.shape[:2]
# Calculate position and make sure the overlay fits within the background
x, y = position
if x + overlay_width > bg_width:
overlay = overlay[:, :bg_width - x]
if y + overlay_height > bg_height:
overlay = overlay[:bg_height - y]
# Split the overlay image into its color and alpha channels
overlay_image = overlay[:, :, :3]
overlay_mask = overlay[:, :, 3:]
# Extract the region of interest (ROI) from the background image
roi = background[y:y + overlay_height, x:x + overlay_width]
# Blend the images
blended = cv2.addWeighted(roi, 1, overlay_image, 0.5, 0, mask=overlay_mask)
background[y:y + overlay_height, x:x + overlay_width] = blended
return background
5. Integrate Everything
Capture video from the webcam and overlay the clothing item based on facial landmarks:
def main():
# Load clothing image with transparency (RGBA)
clothing = cv2.imread('shirt.png', cv2.IMREAD_UNCHANGED)
# Open webcam
cap = cv2.VideoCapture(0)
while cap.isOpened():
ret, frame = cap.read()
if not ret:
break
landmarks = get_landmarks(frame)
if landmarks:
# Example: Use shoulder landmarks (assuming landmark indices 2 and 14)
left_shoulder = landmarks[2]
right_shoulder = landmarks[14]
# Calculate position and size for the clothing overlay
shoulder_width = int(np.linalg.norm(np.array(left_shoulder) - np.array(right_shoulder)))
scale_factor = shoulder_width / clothing.shape[1]
resized_clothing = cv2.resize(clothing, None, fx=scale_factor, fy=scale_factor, interpolation=cv2.INTER_AREA)
# Example: Position clothing at a point between the shoulders
position = (left_shoulder[0], left_shoulder[1] - resized_clothing.shape[0] // 2)
frame = overlay_image(frame, resized_clothing, position)
cv2.imshow('Virtual Dressing Room', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
if __name__ == '__main__':
main()
Explanation
-
Load Libraries and Models: The script starts by loading the necessary libraries and models. Dlib’s pre-trained model is used for facial landmarks detection.
-
Get Landmarks: A function to extract facial landmarks from the detected face.
-
Overlay Image: A function to overlay the clothing image onto the frame. It handles resizing and positioning of the clothing item.
-
Main Function: The main loop captures frames from the webcam, detects facial landmarks, calculates the appropriate position for the clothing item, and overlays it onto the frame. The script continues until the user presses the ‘q’ key.
This is a basic implementation. For a more sophisticated virtual dressing room, you might want to include more advanced features like:
- Body pose estimation for better alignment.
- Different clothing items and accessories.
- Improved blending techniques for a more natural look.
Make sure to adjust the landmark indices and the overlay positioning based on your specific requirements and the clothing items you are using.