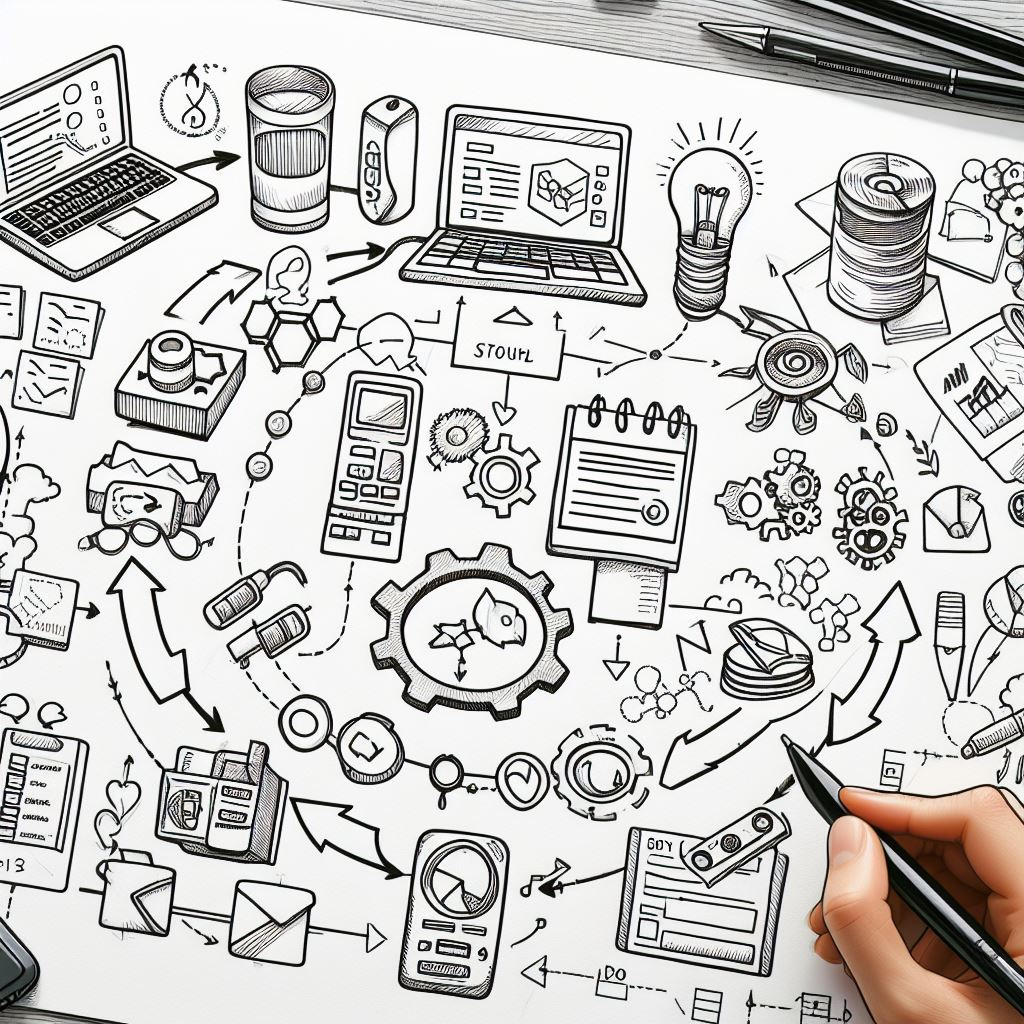
電表度數的識別器
要寫一個識別電表度數的識別器基本的步驟概述:
步驟 1:數據收集
收集包含電表度數的圖片數據。這些圖片應該涵蓋各種電表的類型和不同的光照條件。
步驟 2:數據標註
對收集到的圖片進行標註,標註每張圖片中電表顯示的數字。你可以使用工具如LabelImg來進行圖片標註。
步驟 3:圖像處理
使用OpenCV等圖像處理庫進行預處理,包括:
- 灰度轉換:將彩色圖片轉換為灰度圖片。
- 噪聲去除:應用高斯模糊等技術去除圖片中的噪聲。
- 邊緣檢測:使用Canny邊緣檢測來找到電表上的數字邊界。
- 圖像裁剪:裁剪出包含數字的區域。
步驟 4:光學字符識別 (OCR)
使用OCR技術識別圖片中的數字。Tesseract是一個流行的開源OCR庫,可以用來識別數字。
import cv2
import pytesseract
# 讀取圖像
image = cv2.imread('electric_meter.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 預處理
gray = cv2.GaussianBlur(gray, (5, 5), 0)
edges = cv2.Canny(gray, 50, 150)
# 使用Tesseract進行OCR
custom_config = r'--oem 3 --psm 6 outputbase digits'
digits = pytesseract.image_to_string(edges, config=custom_config)
print("識別出的數字:", digits)
步驟 5:機器學習模型
如果OCR結果不夠準確,可以考慮訓練一個卷積神經網絡(CNN)來進行數字識別。這需要你有標註好的數據集來訓練模型。
- 構建數字識別模型:
import tensorflow as tf from tensorflow.keras import layers, models
構建模型
model = models.Sequential([
layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.Flatten(),
layers.Dense(64, activation='relu'),
layers.Dense(10, activation='softmax')
])
編譯模型
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
訓練模型
model.fit(train_images, train_labels, epochs=5, validation_data=(test_images, test_labels))
2. 使用模型進行預測:
```python
# 預測數字
predicted_digit = model.predict_classes(preprocessed_image)
print("預測的數字:", predicted_digit)
步驟 6:系統集成
將圖像處理和數字識別模型集成到一個完整的系統中,可以用Python Flask或Django來構建一個Web應用,讓用戶上傳電表圖片並返回識別出的數字。
這樣的一個識別器可以用來自動讀取電表度數,提高效率並減少人為錯誤。
Certainly! Here is a complete step-by-step tutorial for creating an electric meter digit recognition system:
Step 1: Data Collection
- Capture Images:
- Collect images of electric meters. Ensure you have a variety of images with different lighting conditions and angles.
- Save these images in a folder, e.g.,
electric_meter_images
.
Step 2: Data Annotation
- Annotate Images:
- Use a tool like LabelImg to annotate the images. Draw bounding boxes around the digits and label them accordingly.
- Save the annotations in a format like Pascal VOC XML or YOLO.
Step 3: Image Preprocessing
-
Install Required Libraries:
pip install opencv-python pytesseract tensorflow
-
Preprocess Images with OpenCV:
import cv2 import numpy as np def preprocess_image(image_path): # Read the image image = cv2.imread(image_path) # Convert to grayscale gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) # Apply Gaussian Blur gray = cv2.GaussianBlur(gray, (5, 5), 0) # Apply Canny Edge Detection edges = cv2.Canny(gray, 50, 150) return edges # Example usage preprocessed_image = preprocess_image('electric_meter_images/example.jpg') cv2.imshow('Preprocessed Image', preprocessed_image) cv2.waitKey(0) cv2.destroyAllWindows()
Step 4: Optical Character Recognition (OCR)
-
Use Tesseract for OCR:
import pytesseract def ocr_image(image): # Custom configuration for Tesseract custom_config = r'--oem 3 --psm 6 outputbase digits' digits = pytesseract.image_to_string(image, config=custom_config) return digits # Example usage digits = ocr_image(preprocessed_image) print("Recognized digits:", digits)
Step 5: Machine Learning Model (Optional for Improved Accuracy)
-
Prepare Dataset:
- Split your annotated images into training and testing datasets.
- Ensure your images are resized to a consistent shape, e.g., 28×28 pixels.
-
Build and Train a Convolutional Neural Network (CNN) with TensorFlow:
import tensorflow as tf from tensorflow.keras import layers, models # Load and preprocess your dataset # Assuming
train_images
,train_labels
,test_images
,test_labels
are available # Normalize the pixel values train_images = train_images / 255.0 test_images = test_images / 255.0 # Build the model model = models.Sequential([ layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)), layers.MaxPooling2D((2, 2)), layers.Conv2D(64, (3, 3), activation='relu'), layers.MaxPooling2D((2, 2)), layers.Conv2D(64, (3, 3), activation='relu'), layers.Flatten(), layers.Dense(64, activation='relu'), layers.Dense(10, activation='softmax') ]) # Compile the model model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy']) # Train the model model.fit(train_images, train_labels, epochs=5, validation_data=(test_images, test_labels)) -
Predict Using the Model:
def predict_digit(image): # Preprocess the image for the model image = cv2.resize(image, (28, 28)) image = np.expand_dims(image, axis=0) image = np.expand_dims(image, axis=-1) image = image / 255.0 # Predict the digit prediction = model.predict(image) digit = np.argmax(prediction) return digit # Example usage digit = predict_digit(preprocessed_image) print("Predicted digit:", digit)
Step 6: System Integration
-
Create a Web Application with Flask:
pip install flask
-
Create the Flask App:
from flask import Flask, request, jsonify import cv2 import numpy as np import pytesseract app = Flask(__name__) def preprocess_image(image_path): image = cv2.imread(image_path) gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) gray = cv2.GaussianBlur(gray, (5, 5), 0) edges = cv2.Canny(gray, 50, 150) return edges @app.route('/upload', methods=['POST']) def upload_image(): if 'file' not in request.files: return jsonify({'error': 'No file uploaded'}), 400 file = request.files['file'] file_path = f'uploads/{file.filename}' file.save(file_path) preprocessed_image = preprocess_image(file_path) digits = pytesseract.image_to_string(preprocessed_image, config=r'--oem 3 --psm 6 outputbase digits') return jsonify({'digits': digits}) if __name__ == '__main__': app.run(debug=True)
-
Run the Flask App:
python app.py
-
Test the Web App:
- Use a tool like Postman to upload images and check the recognized digits.
By following these steps, you will have a complete system that can recognize the digits on electric meters using a combination of image processing, OCR, and potentially a machine learning model for enhanced accuracy.