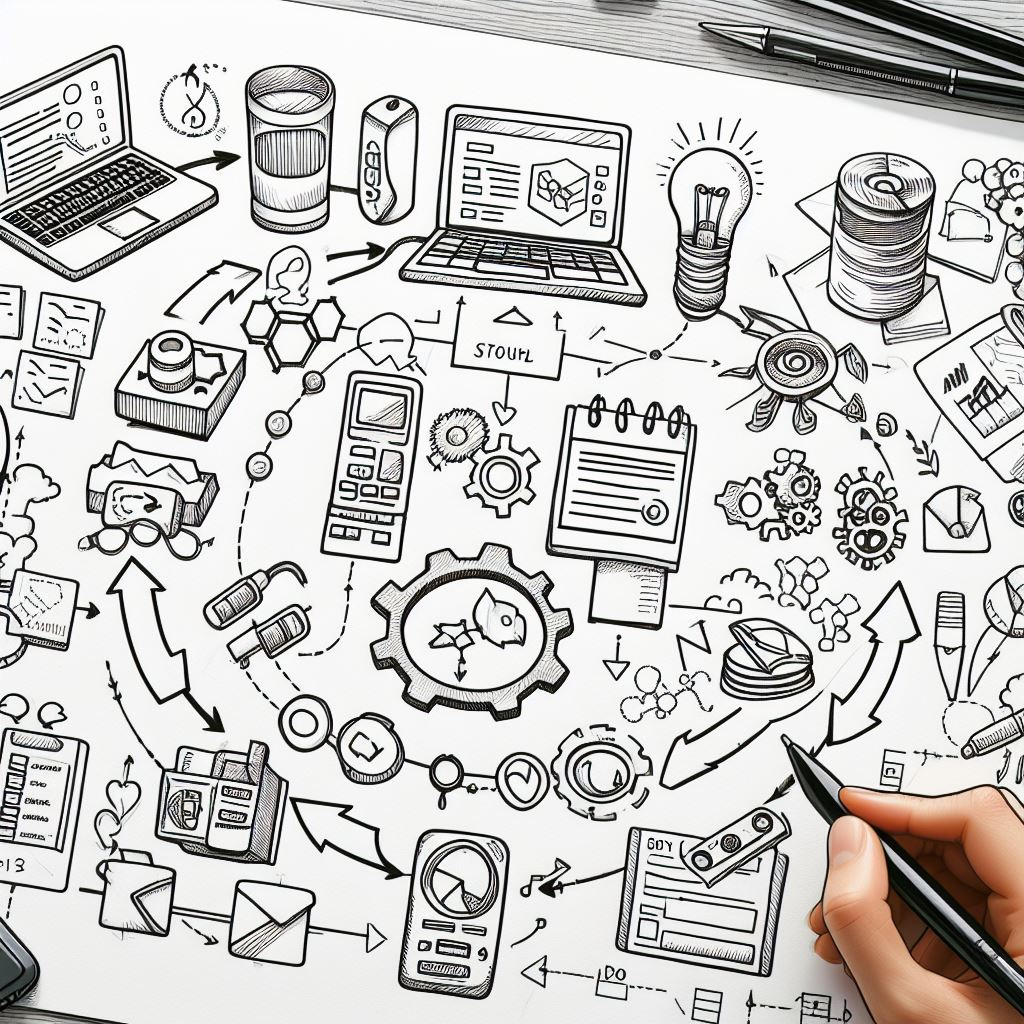
JavaScript的Arrow Function(快箭函數)
JavaScript的Arrow Function(箭頭函數)是ES6引入的一種新的函數表達式,具有簡潔的語法和特定的特性。它們與傳統的函數聲明不同,主要用於簡化函數的寫法,並且在處理this
關鍵字方面有一些獨特的行為。
基本語法
箭頭函數的基本語法如下:
(param1, param2, …, paramN) => { statements }
如果函數只有一個參數,可以省略圓括號:
singleParam => { statements }
如果函數體只有一個表達式,且需要返回值,可以省略大括號和return
關鍵字:
(param1, param2, …, paramN) => expression
各型態的用途與例子
1. 無參數的箭頭函數
當函數不需要任何參數時,可以使用空括號:
const sayHello = () => console.log('Hello');
sayHello(); // 輸出: Hello
2. 單參數的箭頭函數
當函數只有一個參數時,可以省略圓括號:
const square = x => x * x;
console.log(square(5)); // 輸出: 25
3. 多參數的箭頭函數
當函數有多個參數時,需要使用圓括號:
const add = (a, b) => a + b;
console.log(add(3, 4)); // 輸出: 7
4. 帶有函數體的箭頭函數
當函數體內有多行代碼時,需要使用大括號包裹,並顯式使用return
來返回值:
const multiply = (a, b) => {
const result = a * b;
return result;
};
console.log(multiply(3, 4)); // 輸出: 12
5. 返回對象字面量的箭頭函數
當箭頭函數需要返回一個對象字面量時,對象需要用括號包起來,否則會被當作函數體的括號:
const getUser = (name, age) => ({ name: name, age: age });
console.log(getUser('Alice', 30)); // 輸出: { name: 'Alice', age: 30 }
6. 內嵌箭頭函數(高階函數)
箭頭函數可以用於高階函數,如陣列的方法:
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map(n => n * 2);
console.log(doubled); // 輸出: [2, 4, 6, 8, 10]
特性與限制
this
綁定:箭頭函數不會創建自己的this
上下文,它們會捕獲封閉範圍內的this
值作為自己的this
值。因此,箭頭函數適合用在需要保持this
上下文的回調中。
function Person() {
this.age = 0;
setInterval(() => {
this.age++; // `this`正確指向Person對象
console.log(this.age);
}, 1000);
}
const p = new Person();
- 不能用作構造函數:箭頭函數不能用作構造函數(使用
new
運算符),會導致錯誤。
const Foo = () => {};
const foo = new Foo(); // TypeError: Foo is not a constructor
- 沒有
arguments
對象:箭頭函數沒有自己的arguments
對象,可以使用剩餘參數來代替。
const sum = (...args) => args.reduce((acc, val) => acc + val, 0);
console.log(sum(1, 2, 3, 4)); // 輸出: 10
結論
JavaScript的箭頭函數以其簡潔的語法和特定的特性提供了一種編寫函數的新方式,特別是在處理回調和保持this
上下文時非常有用。然而,由於它們的特性與限制,使用時需要謹慎。