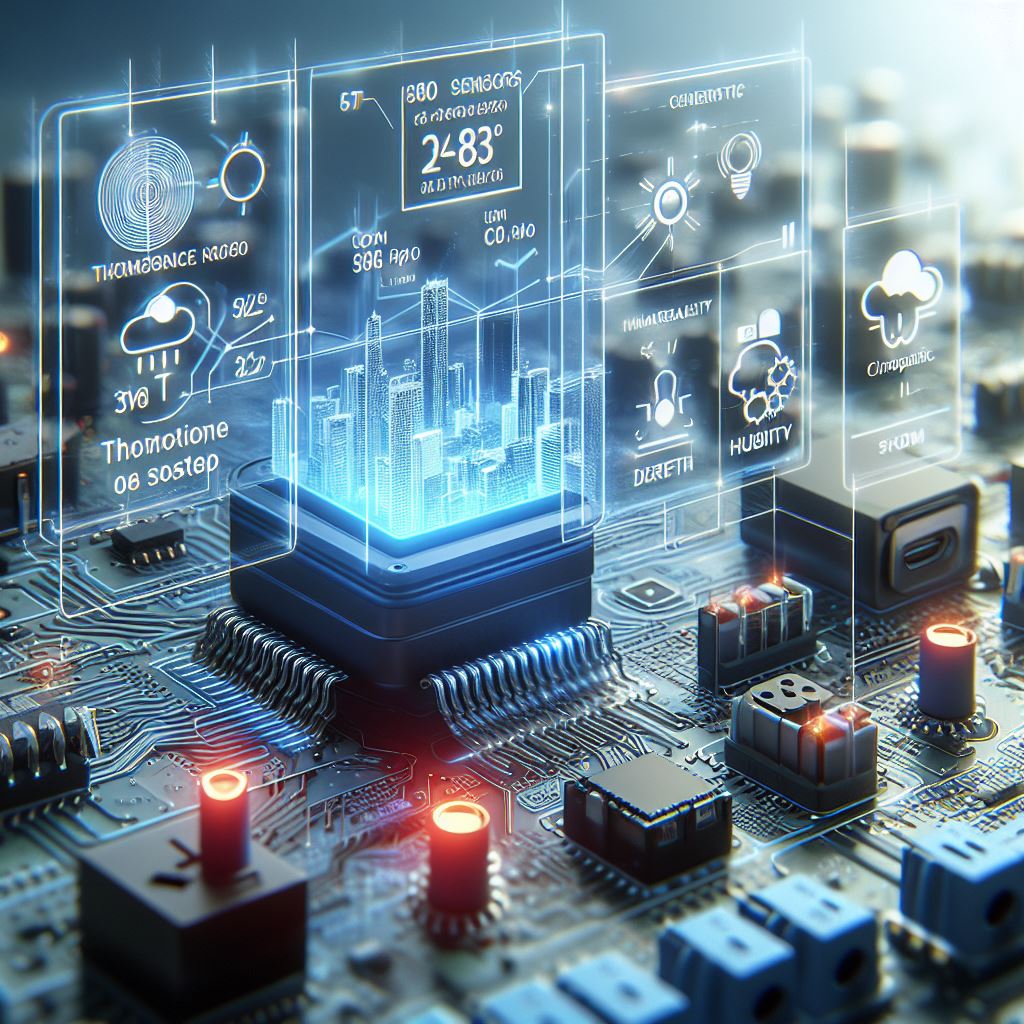
WordPress Custom Post Type(自定義文章型態)
在 WordPress 中,Custom Post Type(自定義文章型態)是一種功能,允許開發者和網站管理員創建和管理與預設的文章(Post)和頁面(Page)不同的內容類型。這些自定義文章類型可以用來組織和展示特定的內容,如產品、活動、作品集、客戶評價等。
以下是一些關於 Custom Post Type 的要點:
- 創建與註冊:使用
register_post_type()
函數來註冊一個新的自定義文章類型。在這個函數中,你可以設定這個文章類型的標籤、公開性、是否支持標籤和類別等選項。function create_custom_post_type() { register_post_type('product', array( 'labels' => array( 'name' => __('Products'), 'singular_name' => __('Product') ), 'public' => true, 'has_archive' => true, 'rewrite' => array('slug' => 'products'), 'supports' => array('title', 'editor', 'thumbnail', 'excerpt', 'comments') ) ); } add_action('init', 'create_custom_post_type');
- 自定義分類法:自定義文章類型可以與自定義分類法(Taxonomies)結合使用,這樣可以更好地組織和分類你的內容。你可以使用
register_taxonomy()
函數來創建自定義分類法,並將它們與特定的文章類型關聯。 - 自定義欄位:你可以為自定義文章類型添加自定義欄位(Custom Fields),這樣可以儲存和顯示更多的特定信息。例如,對於產品文章類型,你可以添加價格、庫存狀態、產品規格等字段。
- 前端顯示:你可以創建自定義模板文件來顯示自定義文章類型的內容。例如,創建
single-product.php
文件來顯示單個產品的詳細信息,或者創建archive-product.php
文件來顯示產品的存檔頁面。 - 查詢與顯示:你可以使用 WP_Query 類來查詢和顯示特定的自定義文章類型。例如,顯示最新的 10 個產品:
$args = array( 'post_type' => 'product', 'posts_per_page' => 10 ); $loop = new WP_Query($args); while ($loop->have_posts()) : $loop->the_post(); the_title(); the_content(); endwhile;
- 管理界面:在 WordPress 後台中,自定義文章類型會顯示在左側菜單中,你可以在那裡添加、編輯和管理這些文章。你還可以自定義管理界面,添加額外的欄位和功能。
實例:建立一個自訂文章類型“product”
這個實例將展示如何使用WordPress的內建函數建立一個自訂文章類型“product”。此自訂文章類型將具有以下屬性:
- 它將在WordPress管理後台中可見。
- 它將有一個專門的歸檔頁面。
- 它將具有以下自訂屬性:
- 產品名稱
- 產品價格
- 產品圖片
- 產品描述
步驟 1:建立自訂文章類型
PHP
register_post_type( 'product', array(
'labels' => array(
'name' => __( 'Products', 'my-textdomain' ),
'singular_name' => __( 'Product', 'my-textdomain' ),
),
'public' => true,
'has_archive' => true,
'supports' => array( 'title', 'editor', 'thumbnail', 'custom-fields' ),
));
步驟 2:建立自訂屬性
PHP
add_action( 'init', 'create_product_meta_fields' );
function create_product_meta_fields() {
add_meta_box( 'product_meta', __( 'Product Details', 'my-textdomain' ), 'display_product_meta_fields', 'product', 'normal', 'high' );
}
function display_product_meta_fields( $post ) {
wp_nonce_field( 'product_meta_nonce', 'product_meta_nonce' );
$product_name = get_post_meta( $post->ID, 'product_name', true );
$product_price = get_post_meta( $post->ID, 'product_price', true );
$product_image = get_post_meta( $post->ID, 'product_image', true );
$product_description = get_post_meta( $post->ID, 'product_description', true );
echo '<table class="form-table">';
echo '<tr>';
echo '<th scope="row"><label for="product_name">' . __( 'Product Name', 'my-textdomain' ) . '</label></th>';
echo '<td><input type="text" id="product_name" name="product_name" value="' . esc_attr( $product_name ) . '" class="regular-text"></td>';
echo '</tr>';
echo '<tr>';
echo '<th scope="row"><label for="product_price">' . __( 'Product Price', 'my-textdomain' ) . '</label></th>';
echo '<td><input type="number" id="product_price" name="product_price" value="' . esc_attr( $product_price ) . '" class="regular-text"></td>';
echo '</tr>';
echo '<tr>';
echo '<th scope="row"><label for="product_image">' . __( 'Product Image', 'my-textdomain' ) . '</label></th>';
echo '<td><input type="file" id="product_image" name="product_image"></td>';
echo '</tr>';
echo '<tr>';
echo '<th scope="row"><label for="product_description">' . __( 'Product Description', 'my-textdomain' ) . '</label></th>';
echo '<td><textarea id="product_description" name="product_description" class="large-text">' . esc_textarea( $product_description ) . '</textarea></td>';
echo '</tr>';
echo '</table>';
}
add_action( 'save_post', 'save_product_meta_fields' );
function save_product_meta_fields( $post_id ) {
if ( ! isset( $_POST['product_meta_nonce'] ) ) {
return;
}
if ( ! wp_verify_nonce( $_POST['product_meta_nonce'], 'product_meta_nonce' ) ) {
return;
}
$product_name = sanitize_text_field( $_POST['product_name'] );
$product_price = sanitize_text_field( $_POST['product_price'] );
$product_image = sanitize_file_name( $_FILES['product_image']['name'] );
$product_description = wp_kses_post( $_