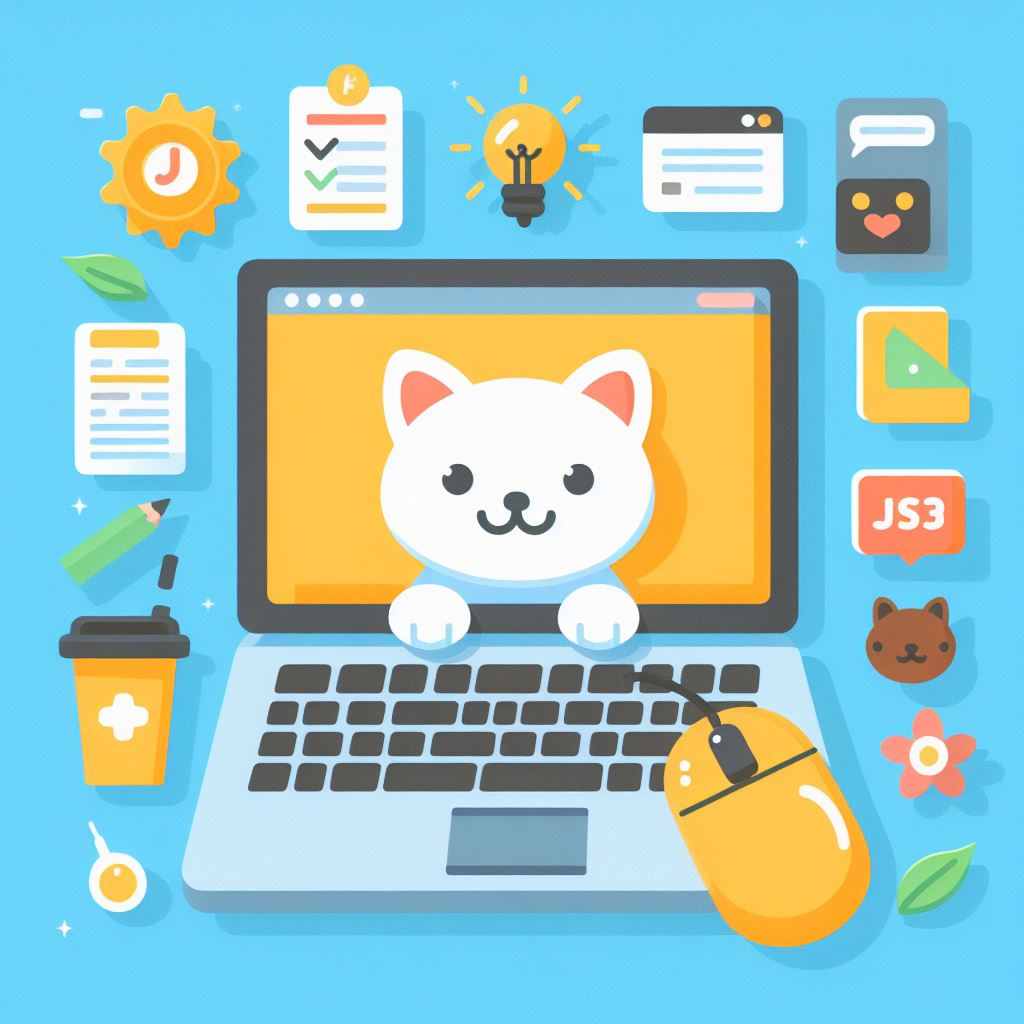
線上衣服換色
線上衣服換色的網頁,使用HTML、CSS和JavaScript來實現。以下示範如何做到這一點:
- HTML部分:用於顯示衣服和選擇顏色的界面。
- CSS部分:用於設置網頁的基本樣式。
- JavaScript部分:用於處理顏色切換的邏輯。
1. HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Online Clothes Color Changer</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<h1>Online Clothes Color Changer</h1>
<div class="image-container">
<img id="clothesImage" src="shirt.png" alt="Shirt">
</div>
<div class="color-picker">
<label for="colorInput">Choose a color:</label>
<input type="color" id="colorInput" name="colorInput">
</div>
</div>
<script src="script.js"></script>
</body>
</html>
2. CSS
/* styles.css */
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #f0f0f0;
margin: 0;
}
.container {
text-align: center;
}
.image-container {
margin-bottom: 20px;
}
#clothesImage {
width: 300px;
height: auto;
}
.color-picker {
margin-top: 20px;
}
3. JavaScript
// script.js
document.addEventListener("DOMContentLoaded", function() {
const colorInput = document.getElementById('colorInput');
const clothesImage = document.getElementById('clothesImage');
colorInput.addEventListener('input', function() {
const color = colorInput.value;
clothesImage.style.filter = `hue-rotate(${getHueRotation(color)}deg)`;
});
function getHueRotation(color) {
// Extract the red, green, and blue components of the color
const r = parseInt(color.slice(1, 3), 16);
const g = parseInt(color.slice(3, 5), 16);
const b = parseInt(color.slice(5, 7), 16);
// Convert RGB to HSL
let max = Math.max(r, g, b), min = Math.min(r, g, b);
let h, s, l = (max + min) / 2;
if (max === min) {
h = s = 0; // achromatic
} else {
const d = max - min;
s = l > 0.5 ? d / (2 - max - min) : d / (max + min);
switch (max) {
case r: h = (g - b) / d + (g < b ? 6 : 0); break;
case g: h = (b - r) / d + 2; break;
case b: h = (r - g) / d + 4; break;
}
h /= 6;
}
return h * 360;
}
});
4. 圖像處理
你需要一個透明背景的衣服圖像,例如 shirt.png
。這個圖像應該是灰色或白色的衣服,這樣可以更好地展示顏色變化效果。
說明
- HTML文件構建基本的網頁結構,包括顯示衣服的圖像和顏色選擇器。
- CSS文件設置網頁的基本樣式,使其更美觀。
- JavaScript文件包含邏輯,用於處理顏色選擇器的輸入並動態改變衣服的顏色。通過改變圖像的
filter
屬性來實現這一點。
這個簡單的實現方式利用了CSS的 hue-rotate
屬性來改變圖像的顏色。當用戶選擇不同的顏色時,JavaScript計算該顏色的色調旋轉角度並應用於圖像。這樣你就可以實現一個簡單的線上衣服換色功能。如果需要更精細的顏色控制和效果,可以考慮使用Canvas API或其他圖像處理技術。